18 min to read
Fizz Buzz
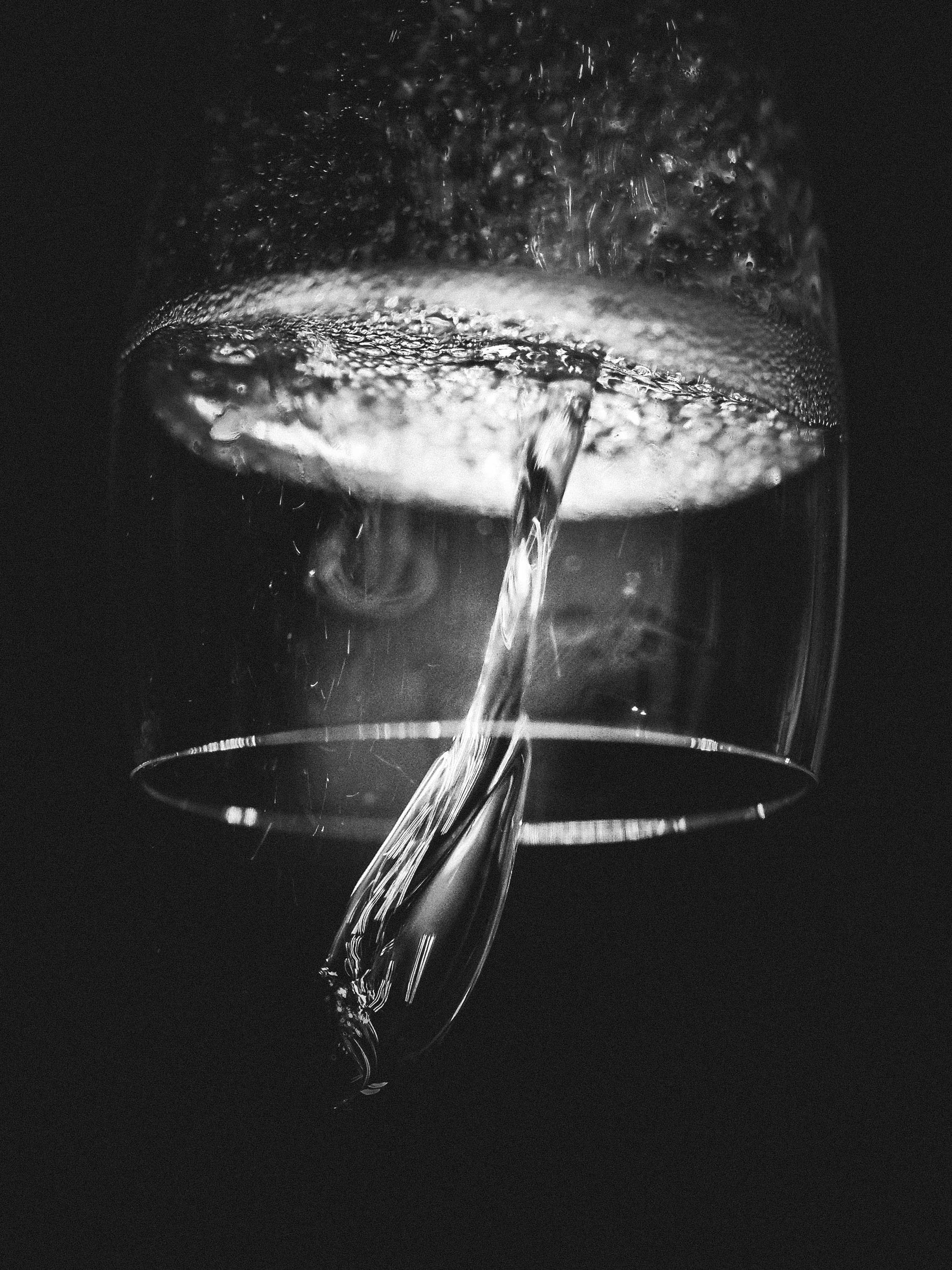
In this article, we bring you a challenge called Fizz Buzz, which was provided by AlgoDaily.
We recommend trying to complete the challenge yourself, before reading our explanation, here.
The Challenge
We’re given a number n
.
Write a function that returns the string representation of all numbers from 1 to n
based on the following rules:
- If it’s a multiple of three, represent it as “fizz”.
- If it’s a multiple of five, represent it as “buzz”.
- If it’s a multiple of both three and five, represent it as “fizzbuzz”.
- If it’s neither, just return the number itself.
As such, fizzBuzz(15)
would result in '12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz'
.
Test Cases
- Expect
fizzBuzz(0)
to equal''
- Expect
fizzBuzz(1)
to equal'1'
- Expect
fizzBuzz(15)
to equal'12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz'
Learn how to complete this challenge using:
The solutions and test cases for this challenge can be found on our GitHub account.
JavaScript
First, we’ll create the fizzBuzz
function and a loop that prints the numbers from 0
to the parameter value n
.
const fizzBuzz = n => {
for (let i = 0; i < n; i++) {
console.log(i)
}
}
fizzBuzz(4) // prints 0, 1, 2, 3
fizzBuzz(10) // prints 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
fizzBuzz(1) // prints 0
We already know, from the test cases above, that this is not the expected behaviour, as the numbers should start from 1
, not 0
.
The loop should also continue until we reach the value of n
, whereas it’s currently stopping one number short (e.g. fizzBuzz(4)
stops at 3
instead of 4
).
We can fix these issues by:
- Assigning the value of
i
to1
in the initialisation statement (let i = 1
). - Amending the condition expression from “
i
is less thann
” (i < n
) to “i
is less than or equal ton
” (i <= n
).
const fizzBuzz = n => {
for (let i = 1; i <= n; i++) {
console.log(i)
}
}
fizzBuzz(4) // prints 1, 2, 3, 4
fizzBuzz(10) // prints 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
fizzBuzz(1) // prints 1
Next, let’s work out if each number is a multiple of three and/or five. To do that, we’re going to use the modulus (%) operator:
const fizzBuzz = n => {
for (let i = 1; i <= n; i++) {
if (i % 3 === 0) {
console.log(`${i} is a multiple of three`)
}
if (i % 5 === 0) {
console.log(`${i} is a multiple of five`)
}
}
}
fizzBuzz(4)
/**
3 is a multiple of three
*/
fizzBuzz(15)
/**
3 is a multiple of three
5 is a multiple of five
6 is a multiple of three
9 is a multiple of three
10 is a multiple of five
12 is a multiple of three
15 is a multiple of three
15 is a multiple of five
*/
fizzBuzz(1)
/**
*/
Now, let’s add a new result
variable which we’ll use to append the correct representation for each number; as per the challenge description:
const fizzBuzz = n => {
let result = ''
for (let i = 1; i <= n; i++) {
if (i % 3 === 0) {
result += 'fizz'
} else if (i % 5 === 0) {
result += 'buzz'
} else {
result += i
}
}
return result
}
console.log(fizzBuzz(4)) // 12fizz4
console.log(fizzBuzz(15)) // 12fizz4buzzfizz78fizzbuzz11fizz1314fizz
console.log(fizzBuzz(1)) // 1
It might look like we’ve finished, but there is one thing missing. See if you can figure out what isn’t right, using the challenge test cases, before we move on.
You should have seen that fizzbBuzz(15)
didn’t return the correct value. The reason is the third challenge requirement:
- If it’s a multiple of both three and five, represent it as “fizzbuzz”.
We’re representing fifteen, which is a multiple of both three and five, as fizz
instead of fizzbuzz
, because we’re not checking if a number matches both conditions. Let’s add that now:
const fizzBuzz = n => {
let result = ''
for (let i = 1; i <= n; i++) {
if (i % 3 === 0 && i % 5 === 0) {
result += 'fizzbuzz'
} else if (i % 3 === 0) {
result += 'fizz'
} else if (i % 5 === 0) {
result += 'buzz'
} else {
result += i
}
}
return result
}
console.log(fizzBuzz(4)) // 12fizz4
console.log(fizzBuzz(15)) // 12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz
console.log(fizzBuzz(1)) // 1
As the last step, let’s make our code more readable, and reduce duplication, by using variables:
const fizzBuzz = n => {
let result = ''
for (let i = 1; i <= n; i++) {
const multipleOfThree = i % 3 === 0
const multipleOfFive = i % 5 === 0
if (multipleOfThree && multipleOfFive) {
result += 'fizzbuzz'
} else if (multipleOfThree) {
result += 'fizz'
} else if (multipleOfFive) {
result += 'buzz'
} else {
result += i
}
}
return result
}
We’ve now completed the FizzBuzz challenge in JavaScript! Keep reading to learn how to complete it using Go.
Go
First, we’ll create the fizzBuzz
function and a loop that prints the numbers from 0
to the parameter value n
.
package main
import "fmt"
func fizzBuzz(n int) {
for i := 0; i < n; i++ {
fmt.Println(i)
}
}
func main() {
fizzBuzz(4) // prints 0, 1, 2, 3
fizzBuzz(10) // prints 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
fizzBuzz(1) // prints 0
}
In the challenge description, we were given an example output of 12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz
, when provided an input of 15.
Notice how the expected output starts at 1
and ends with the value of n
(i.e. 15
or in this case fizzbuzz
).
If we look at what our fizzBuzz
function is printing, we’re starting at 0
and ending one number short of n
.
We can fix these issues by:
- Assigning the value of
i
to1
in the init statement (i := 1
). - Amending the condition expression from “
i
is less thann
” (i < n
) to “i
is less than or equal ton
” (i <= n
).
package main
import "fmt"
func fizzBuzz(n int) {
for i := 1; i <= n; i++ {
fmt.Println(i)
}
}
func main() {
fizzBuzz(4) // prints 1, 2, 3, 4
fizzBuzz(10) // prints 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
fizzBuzz(1) // prints 1
}
Next, let’s work out if each number is a multiple of three and/or five. To do that, we’re going to use the modulus (%) operator:
package main
import "fmt"
func fizzBuzz(n int) {
for i := 1; i <= n; i++ {
if i%3 == 0 {
fmt.Printf("%d is a multiple of three\n", i)
}
if i%5 == 0 {
fmt.Printf("%d is a multiple of five\n", i)
}
}
}
func main() {
fizzBuzz(4)
/**
3 is a multiple of three
*/
fizzBuzz(15)
/**
3 is a multiple of three
5 is a multiple of five
6 is a multiple of three
9 is a multiple of three
10 is a multiple of five
12 is a multiple of three
15 is a multiple of three
15 is a multiple of five
*/
fizzBuzz(1)
/**
*/
}
Now, let’s add a new result
variable which we’ll use to append the correct representation for each number; as per the challenge description:
package main
import "strconv"
func fizzBuzz(n int) {
var result string
for i := 1; i <= n; i++ {
if i%3 == 0 {
result += "fizz"
} else if i%5 == 0 {
result += "buzz"
} else {
result += strconv.Itoa(i)
}
}
}
func main() {
fizzBuzz(4)
fizzBuzz(15)
fizzBuzz(1)
}
Because Go is a strongly-typed language, we have to convert the type of i
from an int
to a string
, using the Itoa
method from the strconv
package, to match the type of the result
variable.
Next, we need to update our fizzBuzz
function to return our result
variable. To do this we add a return type of string
and add the return
statement at the end of the function.
package main
import (
"fmt"
"strconv"
)
func fizzBuzz(n int) string {
var result string
for i := 1; i <= n; i++ {
if i%3 == 0 {
result += "fizz"
} else if i%5 == 0 {
result += "buzz"
} else {
result += strconv.Itoa(i)
}
}
return result
}
func main() {
fmt.Println(fizzBuzz(4)) // 12fizz4
fmt.Println(fizzBuzz(15)) // 12fizz4buzzfizz78fizzbuzz11fizz1314fizz
fmt.Println(fizzBuzz(1)) // 1
}
It might look like we’ve finished, but there is one thing missing. See if you can figure out what isn’t right, using the challenge test cases, before we move on.
You should have seen that fizzbBuzz(15)
didn’t return the correct value. The reason is the third challenge requirement:
- If it’s a multiple of both three and five, represent it as “fizzbuzz”.
We’re representing fifteen, which is a multiple of both three and five, as fizz
instead of fizzbuzz
, because we’re not checking if a number matches both conditions. Let’s add that now:
package main
import (
"fmt"
"strconv"
)
func fizzBuzz(n int) string {
var result string
for i := 1; i <= n; i++ {
if i%3 == 0 && i%5 == 0 {
result += "fizzbuzz"
} else if i%3 == 0 {
result += "fizz"
} else if i%5 == 0 {
result += "buzz"
} else {
result += strconv.Itoa(i)
}
}
return result
}
func main() {
fmt.Println(fizzBuzz(4)) // 12fizz4
fmt.Println(fizzBuzz(15)) // 12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz
fmt.Println(fizzBuzz(1)) // 1
}
As the last step, let’s make our code more readable, and reduce duplication, by using variables:
package main
import (
"fmt"
"strconv"
)
func fizzBuzz(n int) string {
var result string
for i := 1; i <= n; i++ {
multipleOfThree := i%3 == 0
multipleOfFive := i%5 == 0
if multipleOfThree && multipleOfFive {
result += "fizzbuzz"
} else if multipleOfThree {
result += "fizz"
} else if multipleOfFive {
result += "buzz"
} else {
result += strconv.Itoa(i)
}
}
return result
}
func main() {
fmt.Println(fizzBuzz(4)) // 12fizz4
fmt.Println(fizzBuzz(15)) // 12fizz4buzzfizz78fizzbuzz11fizz1314fizzbuzz
fmt.Println(fizzBuzz(1)) // 1
}
Congratulations - we’ve completed the FizzBuzz challenge in Go! Why not try and solve it using JavaScript too?
Modulus (%) operator
The modulo operation finds the remainder after division of one number by another.
You’d be forgiven if you’re still none the wiser about what it means, that “official” explanation isn’t exactly clear, so let’s look at some examples.
Let’s start with some simple division; what is eight divided by four (8 / 4
)? That’s easy - 2 - because four goes into eight twice.
What is ten divided by four (10 / 4
)? This time our result is a fraction, 2.5, because four doesn’t go into ten an exact number of times.
Another way of answering ten divided by four, is four goes into ten twice (8
) with a remainder of two (10 - 8 = 2
). And that’s exactly what modulus is; it returns the remainder, so ten modulus four (10 % 4
) is two.
How can this help us with Fizz Buzz? Well, if we need to find the numbers which are divisible by three and/or five, we can check for the modulus/remainder value.
For example, to check whether a number is divisible by three:
1 % 3
- three goes into one zero times, so the remainder is one (1 - 0 = 1
).2 % 3
- three goes into two zero times, so the remainder is two (2 - 0 = 2
).3 % 3
- three goes into three one time, so the remainder is zero (3 - 3 = 0
).4 % 3
- four goes into three one time, with a remainder of one (4 - 3 = 1
).
Notice how, when the number is divisible by three, the remainder is zero. Therefore, we can say when the remainder is zero the number is a multiple of three.
If anything in this article wasn’t clear, feel free to reach out to us!
Comments